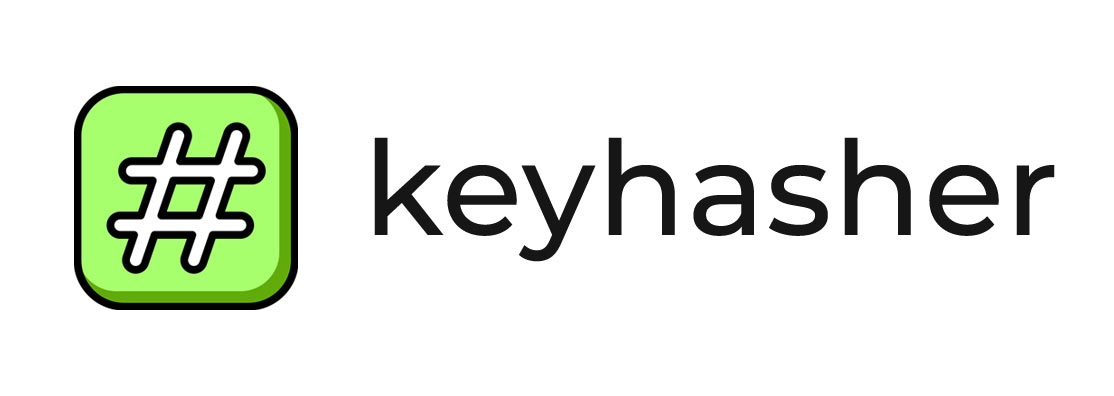
Simple, effective, easy to implement hashing for JavaScript, with KeyHasher
const { encrypt, decrypt, hash, hashCompare } = require('keyhasher');
const passCode = process.env.PASS_CODE || 572; // set passCode in .env or use directly
var encryptedWord = encrypt('passwordInput', passCode);
console.log(`Encrypted Phrase: ${encryptedWord}`) // Output: "QGFkX1NeEkVXYGZXYFVX"
var rawWord = decrypt(encryptedWord, passCode);
console.log(`Output: ${rawWord}`) // Output: "Normal Sentence"
const hashed = hash(encryptedWord); // encryption + hashing
console.log(`Hashed: ${hashed}`);
// Output: "5bf22a00c0df8757f68e700066bd56c5edffc4103f9587666ccbde062a0f52f5"
const isCorrect = hashCompare(hash('user given password'), hashed);
// it hashes user input And compares with the hashed value stored in database
console.log(isCorrect); // returns a boolean
Last months download: